Getting Started with Reteno Plugin for Android
- Install cordova-plugin-reteno using
cordova
:
cordova plugin add cordova-plugin-reteno
- Install
firebase
plugin in application:
cordova plugin add cordova-plugin-reteno-firebase
Setting up SDK
Follow our setup guide to integrate the Reteno SDK with your app.
Step 1: Add Android platform
cordova platform add android
Step 2: Set up your Firebase application for Firebase Cloud Messaging:
-
Download your
google-services.json
config file (see how here). -
Add the above file to your root
app/
folder.
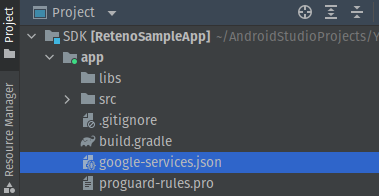
- Copy your FCM Server Key. In the Firebase console, click the gear icon next to Overview.
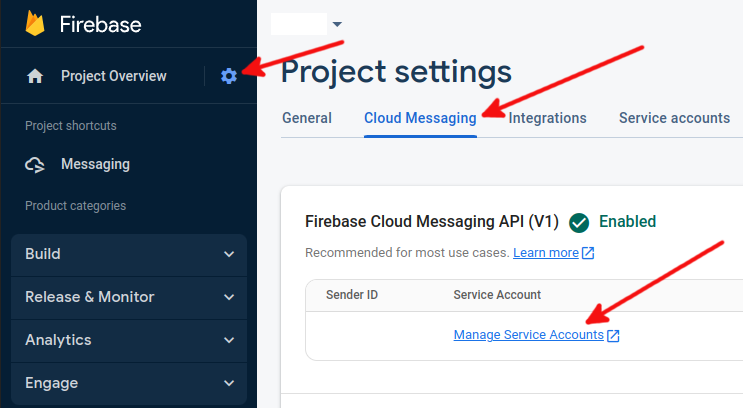
Then click Project Settings->Cloud Messaging -> Manage Service Accounts.
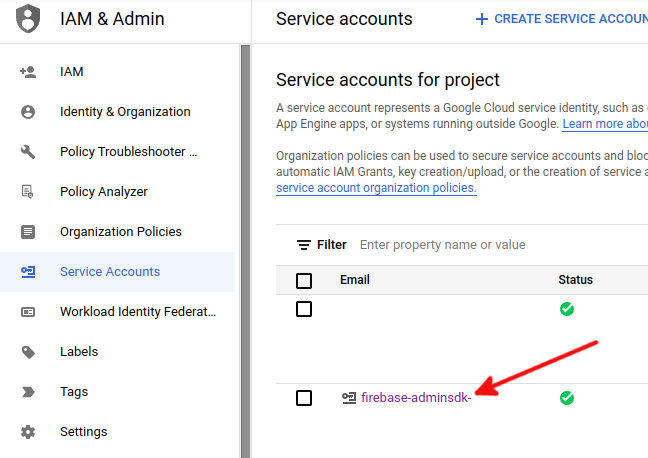
Go to Service accounts to download FirebaseAdminSdk account's json key.
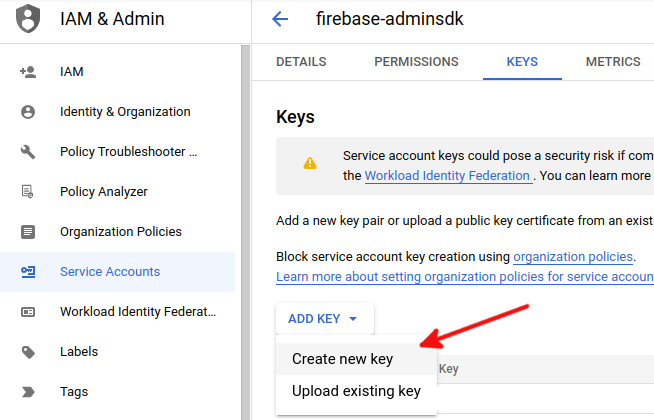
- Follow this manual to set up Yespo admin panel with your Firebase key.
Now you are ready to run your app and send a marketing push notification to your application.
Step 3: Edit your MainApplication class
Below is sample code you can add to your application class which gets you started with RetenoSDK
.
package [com.YOUR_PACKAGE];
import android.app.Application;
import androidx.annotation.NonNull;
import com.reteno.core.Reteno;
import com.reteno.core.RetenoImpl;
import com.reteno.plugin.CordovaRetenoApplication;
public class MainApplication extends Application implements CordovaRetenoApplication {
private Reteno retenoInstance=null;
@Override
public void onCreate() {
super.onCreate();
retenoInstance = new RetenoImpl(this, "YOUR_API_KEY");
}
@NonNull
@Override
public Reteno getRetenoInstance() {
return retenoInstance;
}
}
Step 4: Link development plugin folder (optional):
In case you have a local copy of Reteno Cordova Plugin, you may want to add it to the project, in order to be able to modify or debug it. If this is the case, you should remove the current plugin version from npm and add the local one. Provided that the plugin is located at the parent folder, this can be done as follows:
cordova plugin remove cordova-plugin-reteno
cordova plugin add --link <path_to_plugin_folder>/cordova-plugin-reteno/
cordova plugin remove cordova-plugin-reteno-firebase
cordova plugin add --link <path_to_plugin_folder>/cordova-plugin-reteno-firebase/
So, config.xml
file should contain one line like this:
<plugin name="cordova-plugin-reteno" spec="file:../cordova-plugin-reteno" />
<plugin name="cordova-plugin-reteno-firebase" spec="file:../cordova-plugin-reteno-firebase" />
Step 5: Firebase usage
If you already use Firebase for Remote notifications or would like to use Firebase along with Reteno, you can use it as it is, because cordova-plugin-reteno-firebase provides Firebase support out of the box.
Run Android version
- Run from command line:
cordova run android
- Run from Android Studio: Go to plaftforms/android folder. Create android studio project and run
MainActivity
class
Run your app on a physical Android device to make sure it builds correctly.
Note
Android Debug Mode enables you to ensure that all events and user properties are logged correctly.